iOS SDK User’s Guide#
Introduction#
The Rave iOS SDK provides a complete solution to adding beautiful personal and social experiences to your mobile games.
Running and Using the Demo App#
If your distribution does not include a demo project your scene pack will also serve as your demo. Simply run the Demo target.
Integrating Rave SDK into Your XCode Project#
Tip
For the best development experience and compatibility, always use the latest version of Xcode.
Step 4: Add Required System Frameworks#
The Rave Social SDK depends on several Apple-provided frameworks. To add these to your project:
In the project navigator, select your project.
Select your app target.
Click on the General tab.
Scroll down to the Frameworks, Libraries, and Embedded Content section.
Click the + button.
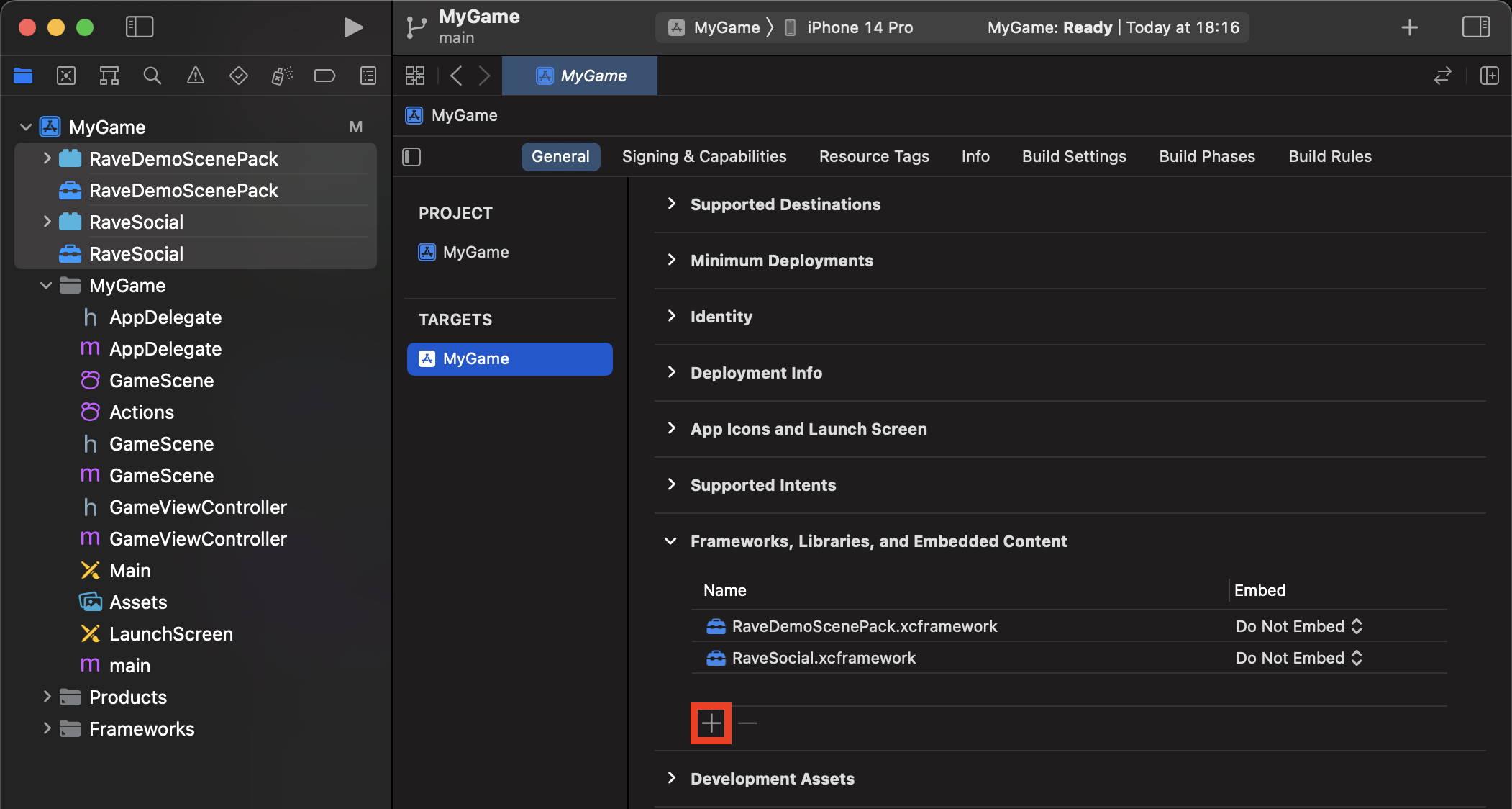
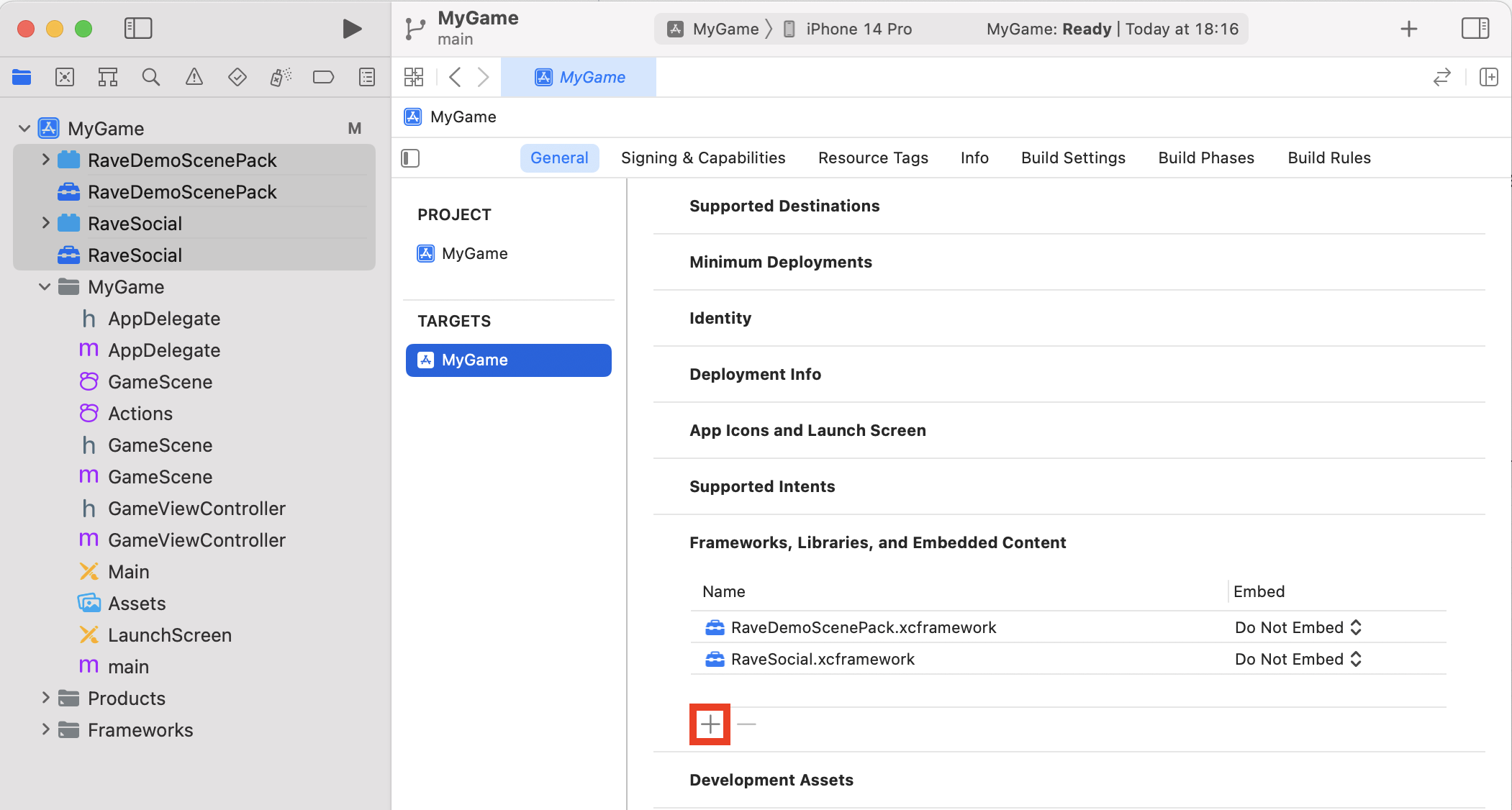
A view with your bundled frameworks will appear. Add the following libraries and frameworks:
Accounts.framework
AddressBook.framework
AdSupport.framework
AssetsLibrary.framework
CFNetwork.framework
CoreData.framework
CoreGraphics.framework
CoreLocation.framework
CoreMotion.framework
CoreText.framework
GameKit.framework
iAd.framework
libsqlite3.dylib
libxml2.dylib
libz.dylib
MediaPlayer.framework
MessageUI.framework
MobileCoreServices.framework
QuartzCore.framework
SafariServices.framework
Security.framework
Social.framework
SystemConfiguration.framework
Attention
Adding Third-Party Frameworks – If your app utilizes features that relies on third-party frameworks, you must ensure that they are included in your project. We recommend using a package manager such as CocoaPods to add these frameworks efficiently. CocoaPods simplifies the process of managing dependencies and ensures that all required libraries are correctly included in your project.
Here is a brief overview of the steps to add a third-party framework using CocoaPods:
Install CocoaPods if it is not already installed.
Set up CocoaPods in your project.
Add the required third-party frameworks to your Podfile.
Install the pods and open the generated .xcworkspace file in Xcode.
For detailed instructions on how to use CocoaPods, please refer to the CocoaPods Getting Started guide.
For integration with specific social platforms, add the following dependencies to your Podfile:
Google Sign-In: If your app utilizes Google Sign-In, add the following line to your Podfile:
pod 'GoogleSignIn'
Facebook SDK: If your app utilizes Facebook, add the following lines to your Podfile:
pod 'FBSDKCoreKit' pod 'FBSDKLoginKit' pod 'FBSDKShareKit'
Warning
Missing third-party frameworks can cause runtime crashes and integration issues. Double-check that all necessary frameworks are included in your project and are properly configured.
Step 5: Add Linker Flags#
Click the root project node in the “Project Navigator”
Click the “Build Settings” tab
Ensure “All” is selected
Search for “Other Linker Flags”
Double-click the right side of the table view row
Add
-ObjC
to the linker flags
Step 6: Edit Your Project’s .pch file#
Open your project’s .pch file (usually named ProjectName-Prefix.pch), and add the following to it:
#ifdef __OBJC__
#import <RaveSocial/RaveSocial.h>
#endif
Step 7: Edit Your Project’s Target Properties#
Add the following keys to your Project’s “Target Properties” in the “Info” tab with their corresponding values (see the demo for example usage):
FacebookAppID must be set to the appropriate value as the Facebook SDK internally refers to it by this name
FacebookClientToken must be set with the value found under Settings > Advanced > Client Token in your Facebook App Dashboard
The URLScheme for Facebook which must match the FacebookAppID provided in the JSON config (with “fb” prepended)
The URLScheme for Google Identity which must match the inverse of the Google client ID.
The URLScheme which must match the bundle ID for your app
Be sure your bundle identifier matches the identifier you register with Facebook, and Google
New in SDK 3.4.2: Required plist key/value pairs to account for iOS 10 changes#
NSContactsUsageDescription - String value will be shown when prompting user permission to access phonebook contacts (i.e. “Contacts will be used to find in-game friends”). If absent application will crash when attempting to access contacts
NSCameraUsageDescription - String value will be shown when prompting user for permission to access the camera (i.e. “Camera will be used to createa profile picture”). If absent application will crash when attempting to access the camera
NSPhotoLibraryUsageDescription - String value will be shown when prompting user for permission to access their photo library (i.e. “Photo library is used for profile pictures”). If absent application will crash when attempting to access the photo library
New in SDK 3.4.2: Required iOS setting when RaveSettings.General.AutoCrossAppLogin is enabled#
RaveSettings.IOS.ApplicationGroupIdentifier - identifier for application group to be used for sharing Cross-application Login data between apps. Must be application group specified in application entitlements for the integrating app. Required when Cross-application Login is enabled.
Step 8: UIApplicationDelegate Window Property Requirement#
UIApplicationDelegate
has an optional window property starting in iOS 5. The Rave Social iOS client uses this window
to display some overlays. Please ensure your application delegate implements the window property. Failure to ensure
window creation and access will result in a missing selector and the client will not be able to run.
// Declaring the property in your app delegate interface will cause
// Xcode to create the methods for you:
@property (nonatomic, strong) UIWindow * window;
Initializing the SDK#
Tip
If Rave does not initialize, check the log for exception messages about invalid or missing settings. See the SDK Logging section for more information on logging.
Integrate the SDK into your application by adding the following code to your app delegate’s
-application:didFinishLaunchingWithOptions:
method:
// imports to expose necessary functionality
// Set application delegate window property prior to calling
self.window = someWindow;
// Init will be the following
[BigFishScenePack initializeScenePack];
If you’re not using the scene pack, call [RaveSocial intializeRave]
or one of its variants instead.
If your application uses Facebook SDK 4.x for best results call initializeRave or initializeScenePack that includes launch options from your app delegate’s -application:didFinishLaunchingWithOptions:
method.
New integration Step
There is a new integration step for RaveSocial: in your application: From your app delegate’s - application:openURL:sourceApplication:annotation:
method call RaveSocial +handleURL:sourceApplication: annotation:
returning YES if Rave automatically handled the URL.
Settings Priority and Overrides#
Note
You must specify a Rave application key to configure the Rave SDK. You can create Rave applications and view their keys in the Rave Developer Portal. See the Creating an Application section in the portal documentation if you have not yet created a Rave application.
Settings can be specified in a number of ways. The priority of settings, from highest to lowest is:
Programmatic settings (
RaveSettings
class)App .plist
JSON (
config.json
file)
This gives you the ability to have a core configuration that works on multiple platforms, where you can override keys per platform either in code or platform specific config (plist).
Attention
Rave will terminate your application with an exception if one or more required settings are missing
Default app configuration data is expected to be in a file in the application bundle by the name config.json
. Here
is an example/template:
{
"RaveSettings.General.ServerURL":"<rave server url including https>",
"RaveSettings.General.ApplicationID":"<rave application id>",
"RaveSettings.General.ConfigUpdateInterval": "3600",
"RaveSettings.General.ContactsUpdateInterval": "21600",
"RaveSettings.Facebook.ApplicationId":"<Facebook application id>",
"RaveSettings.General.AllowForceDisconnect": false,
"RaveSettings.Facebook.ReadPermissions": "public_profile,email",
"RaveSettings.General.AutoCrossAppLogin": true,
"RaveSettings.General.AutoGuestLogin": true,
"RaveSettings.General.ThirdPartySource": "bigfishgames",
"RaveSettings.General.AutoInstallConfigUpdates": true,
"RaveSettings.General.LogLevel" : "Error"
}
To initialize RaveSocial with a different JSON file, add the following to your initialization code:
NSURL * configURL; // should be resolved to some URL that you define
[BigFishScenePack initializeScenePackWithConfig:configURL];
Available Settings#
Common Settings Between SDKs#
Note
Required settings are highlighted in bold in the table below.
Setting Key |
Description |
Default |
---|---|---|
RaveSettings.General.ApplicationID |
Rave Application ID |
|
RaveSettings.General.ServerURL |
Rave Server URL, start with https:// |
|
RaveSettings.General.LogLevel |
Rave Log level, “quiet, error, warn, info, verbose, debug” are valid options |
“error” |
RaveSettings.General.AutoSyncInterval |
Rave automatic configuration sync interval in seconds, 0 = 0ff, valid {3600-86400} |
21600 |
RaveSettings.General.AutoGuestLogin |
Automatic guest login |
true |
RaveSettings.General.AutoCrossAppLogin |
Automatic cross-app login |
true |
RaveSettings.General.AutoInstallConfigUpdates |
Automatically install configuration updates after download |
true |
RaveSettings.General.AllowForceDisconnect |
Allow resolving connect conflicts by forceably disconnecting previous account is allowed |
false |
RaveSettings.General.NetworkTimeout |
Network timeout in ms, valid range is 0-60000 |
30000 |
RaveSettings.General.ContactsUpdateInterval |
How often to update contacts in seconds, 0 = off, 3600-86400 is valid range |
21600 |
RaveSettings.General.ConfigUpdateInterval |
How often to check for configuration updates, 0 = off, 3600-86400 is valid range |
21600 |
RaveSettings.General.ThirdPartySource |
If integrating with a supported third-party system, what the source key is |
|
RaveSettings.General.DefaultResourcesPath |
Default resources path |
|
RaveSettings.General.AutoMergeOnConnect (read-only) |
Automatically merge users if there is a conflict |
false |
RaveSettings.General.AutoSyncFriends |
Comma separated list of social providers for which friends will be automatically synced. |
Facebook, Google, Phonebook |
RaveSettings.Facebook.ApplicationId |
Rave Facebook Application ID |
|
RaveSettings.Facebook.ReadPermissions |
Comma separated list of Facebook read permissions (e.g. public_profile,email…) omitting spaces. Please refer to Facebook documentation for further details |
public_profile, email, user_friends |
RaveSettings.Facebook.AlwaysUseLiveContacts |
If Facebook contacts should always be kept current |
false |
RaveSettings.Google.ClientID |
Google Client ID |
|
RaveSettings.Google.BackendClientID |
The web Google client ID configured in the portal |
|
RaveSettings.Google.ReadPermissions |
Comma separated list of Google read permissions (e.g. email,contacts…) omitting spaces. Please refer to Google documentation for further details |
email,contacts |
RaveSettings.DataMirror.Achievements |
Plugins to enable achievements mirroring for |
|
RaveSettings.DataMirror.Leaderboards |
Plugins to enable leaderboards mirroring for |
iOS-only Settings#
Setting Key |
Description |
Default |
Required |
---|---|---|---|
RaveSettings.IOS.ApplicationGroupIdentifier |
application group identifier for sharing user data |
||
RaveSettings.IOS.InitGameCenterOnStartUp |
If GameCenter should initialize on start-up |
||
RaveSettings.IOS.BundleName |
Bundle name |
There are constants available with names the same as the keys (e.g. RaveThirdPartySource is the constant for the matching key).
Built-In Big Fish Game Personalization Experiences#
The BFC SDK comes equipped with a set of pre-built Big Fish Games experiences. They can be easily and quickly integrated into any Big Fish mobile game to provide a consistent experience for basic game personalization features like Player Login, and Player Profile Management.
Although these experiences by default reflect the look and feel of the Big Fish brand, they can be customized to fit the look and feel of individual games by changing the skin and layout of individual features.
See the Personalizing the Built-in Experiences section to find out how to personalize these experiences.
Player Login Experience#
The default Login experience allows the player to log in, and create a new gamer account via any of these methods:
Sign up with a new email address
Sign in with an email address associated with player’s Big Fish ID account
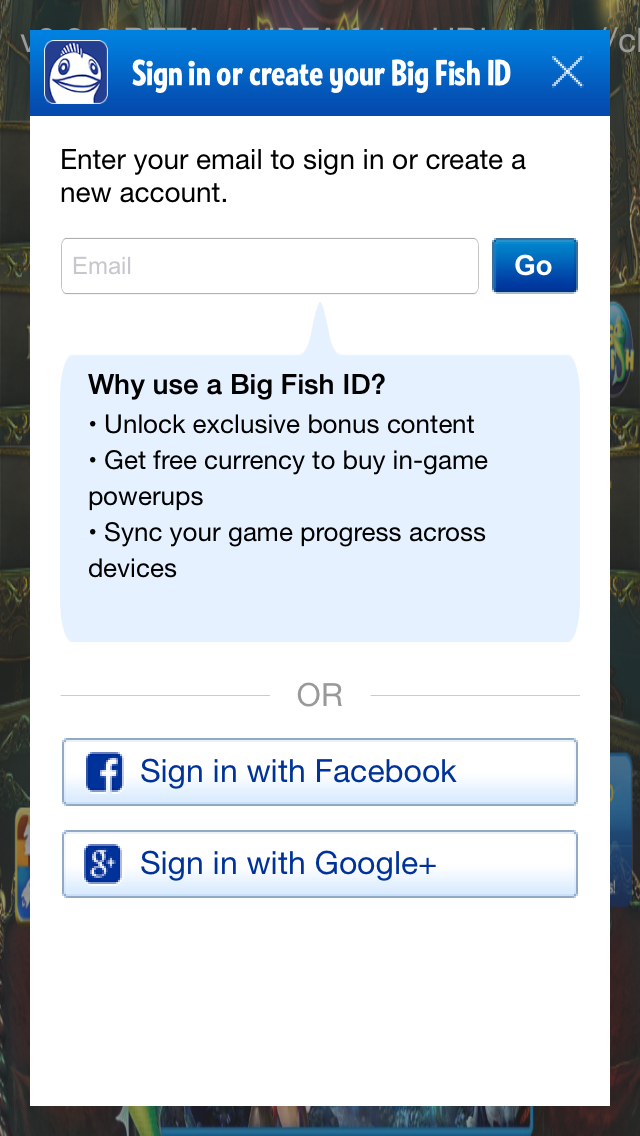
In order to integrate this scene it’s important that the Facebook and Google apps used in the Rave integration are properly configured. Please see Integrating Third Party Authentication section for instructions on how to configure the social networks apps.
Sign up data is somtimes returned in certain regions for new users. See Getting COPPA or CASL Information from User Sign Up section for more on this.
To integrate the sign-up experience:
RaveSignUpEmailScene * signUpScene = [RaveSignUpEmailScene scene];
signUpScene.signUpCallback = ^(RaveCallbackResult result, BigFishSignUpData * signUpData, NSError * error) {
if (result == RaveResultError) {
// handle error
} else if (result == RaveResultCanceled) {
// handle canceled
} else if (result == RaveResultSuccessful) {
if (signUpData) {
// handle the sign up data if needed (canada, us)
} else {
// there is no sign up data returned for other regions
}
}
};
[signUpScene show];
To integrate the sign-in experience:
RaveLoginScene * loginScene = [RaveLoginScene scene];
loginScene.signUpCallback = ^(RaveCallbackResult result, BigFishSignUpData * signUpData, NSError * error) {
// same as above
};
[loginScene show];
Registering and Handling Login Callbacks#
RaveSocial provides key Scene and Login status callbacks that a developer can register and listen to.
Login Status Notification#
The general Login Callback (not to be confused with the RaveLoginScene
callback) is used to notify the developer
that a login status change of some kind has occured. This is useful because it may indicate that some social features
are now available.
[RaveSocial setLoginStatusCallback:^(enum RaveLoginStatus status, NSError * error) {
if (status == RaveLoginError) {
// respond to the error condition
} else if (status == RaveLoggedIn) {
// respond to loggedIn status
// at thish time you may also check different fields of the current user
// by accessing RaveSocial.usersManager.current
} else if (status == RaveLoggedOut) {
}
}];
Directly Logging In to Authentication Providers#
If using the built-in Big Fish Login experiences is not desired, developer can build completely custom login experience using Rave’s login APIs.
Using our loginWith
API, you can directly invoke a login via Facebook or Google. Please use the following
constants:
Facebook
Google
[RaveSocial loginWith:@"Facebook" callback:^(NSError * error) {
// result handler
}];
Checking Readiness of Authentication Providers#
Just like with the direct login API - you can also see if an authentication provider is ready to be used. “Readiness” indicates
that a user has connected this social account to their Rave Social account and also that the token or local
authentication for this social account was successful. Call convenience functions in RaveSocial
to check a plugin’s
readiness. If a plugin is not ready but you desire a ready status, use the matching connect methods in RaveSocial
to establish a connection with the the desired plugin.
[RaveSocial checkReadinessOf:@"Facebook" callback:^(BOOL isReady, NSError * error) {
// handle result
}];
// connecting to Facebook if it fails a readiness check
[RaveSocial connectTo:@"Facebook" callback:^(NSError * error) {
if (error) {
// handle error
} else {
// success
}
}];
Player Profile Management Experience#
The profile management experience screen allows players to build out their game profile by adding a profile picture, email, and display name, as well as connecting various social networks to connect with friends.
This screen will also have a link to login or sign-up if the user hasn’t done so already.
To integrate the Profile Management experience:
RaveAccountInfoScene * accountInfoScene = [RaveAccountInfoScene scene];
accountScene.signUpCallback = ^(RaveCallbackResult result, BigFishSignUpData * signUpData, NSError * error) {
// handle any login or sign-up actions here
};
[accountInfoScene show];
Find Friends Experience#
The Find Friends screen allows the player to connect their Facebook and Google accounts, and their local Phonebook to find friends who are also playing Big Fish games.
As the player connects his social network account to his gamer account, Rave will keep track of which of player’s friends are also playing Big Fish games and will add those friends to the list of player’s gamer contacts. These friends will be available in games for in-game interactions such as sending gifts to them and for social leaderboards.
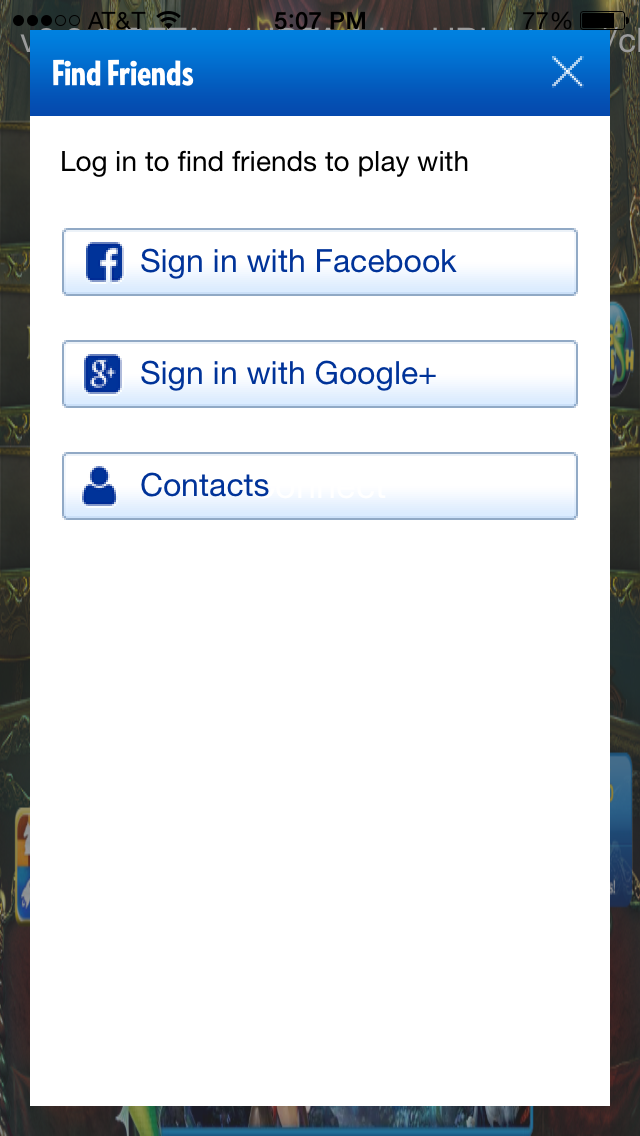
To integrate the Find Friends experience:
RaveFindFriendsScene * findFriendsScene = [RaveFindFriends scene];
[findFriendsScene show];
Getting COPPA or CASL Information from User Sign Up#
If you are in the US region and need information about a new user’s COPPA compliance, or are in the Canadian region and need information about whether the user has accepted newsletter sign-up, this data can be checked by setting a callback on RaveAccountInfoScene
, RaveLoginScene
and RaveSignUpEmailScene
. Note that signUpData
can be returned as nil in certain situations.
The newsletter name for sign up can be set in the setting BigFishSettings.General.NewsletterName
An example usage:
RaveAccountInfoScene * accountScene = [RaveAccountInfoScene scene];
accountScene.signUpCallback = ^(RaveCallbackResult result, BigFishSignUpData * signUpData, NSError * error) {
if (result == RaveResultError) {
// handle error
}
if (signUpData == nil) {
// no sign up data (maybe it was cancelled)
} else {
// For Canada:
// signUpData.acceptedNewsletter tells you if the user opted-in to receive newsletter
// For US:
// signUpData.passedCoppaCheck tells you if the user's age is COPPA Compliant
// signUpData.birthYear will contain the user's year of birth
}
};
[accountScene show];
Personalizing the Built-in Experiences#
The built-in Big Fish game experiences reflect the look and feel of the Big Fish brand, but they can be customized to fit the look and feel of individual games by changing the skin and layout of individual components. The look and feel of the screens can be changed by modifying the resource files that were used to build the screens.
The Rave SDK UI framework allows for native Android and iOS UI to be generated using XML- and CSS-based UI definitions. An individual screen (called a Scene in the SDK) is a combination of XML and native code used to lay out and provide UI functionality. Scenes are generally used for full-screen or dialog-based UI layouts, such as Login or Account Management. Widgets are smaller UI building blocks, such as a button with specialized behavior.
Every built-in experience has at least one XML layout and at least one controlling class, called the Scene class. The Scene class is native code.
Scene Resources#
The scene XML can refer to resources in the local app configuration, such as images, fonts or other XML. Resources can be redefined dynamically via an app configuration bundle and distributed live using the Rave backend service. The default location for resources on Android is in the assets folder of the game or app. The default location in iOS is in the Scene Pack’s resource bundle. Both platforms can share the same resources.
Changing the Look and Feel#
In order to change the color scheme, the skin or individual text or images used in the creation of the built-in experience, the individual assets need to be created and replaced in the exact file structure used in the project. Once replaced the project needs to be restarted for changes to take effect.
Attention
It is important the file structure stays intact so that the SDK and the logic in the Scene class are able to find the assets. Failing to do so may result in an error or an app crash.
Changing Layout#
Since all UI elements in the built-in experiences are dynamically created from XML and CSS scene resource descriptions, only the XML and CSS files need to be modified to change the screen layout. Similarly to the look and feel changes, the project needs to be restarted for those changes to take effect.
Changing Functionality#
If using the built-in Big Fish game experiences is not appropriate for a game, you can build completely custom game UI by using Rave’s data APIs. These APIs expose all functionality available through the Rave system and are used to build the built-in experiences.
For example, instead of integrating the built-in Login screen, you can build a custom login UI by using Rave’s login APIs.
Remotely Updating the Look and Feel of the Experience#
Since all UI elements used in the built-in experiences are dynamically created from resource files, by changing the resource files the look and feel can be modified. Rave supports remote upgrades of these resources. The Rave SDK regularly queries the backend service to check for updated resource files. Resource bundles with new contents can be uploaded through the Rave Developer Portal.
To update UI resource files remotely:
Copy the /resources folder included in the project (and the SDK distribution) to another location where it can be modified safely. Make appropriate changes to individual asset files, CSS and XML files without changing their location.
Once done making changes, create a zip archive from the resources folder called resources.zip.
Upload the file to the Rave Developer Portal. See REF GOES HERE for instructions.
Users and Identities#
At the core of Rave’s game personalization is the concept of a player’s gamer account. This account reflects the gamer identity of an individual person who maybe be playing several Big Fish games across many different devices, including iPad, iPhone, Android phones, and Android tablets.
Every Big Fish gamer account has a globally unique Rave ID, a 32-digit hex number, which can be used to uniquely identify the player for game logic purposes. This Rave ID is created when the player first begins playing a Big Fish game, even if they have not logged in with an explicit authentication method such as Facebook. We will refer to a user that has a Rave ID, but no authentication or personalized data associated with their account as an anonymous user. This Rave ID is persisted for the lifetime of that player’s account.
Later as the player continues to build out their gamer profile by adding a display name or email address to their account, the player will move to the “Personalized” state.
Once the player logs in with any of the available authentication methods, they will move to the “Authenticated” state. Today the available methods of authentication are Big Fish ID, Facebook and Google.
As the player plays more Big Fish games across more devices and continues to build out their profile by connecting additional social networks and additional pieces of personal information (e.g. a profile picture), these attributes are attached to their Rave ID and they can be accessed via the User API. The figure below gives a logical representation of a player’s account and all attributes associated with it.
Attribute |
Description |
---|---|
UUID |
A 32-digit hex number that acts as the Rave ID for the user. It is assigned when a user is created and is immutable. Note that although the value is immutable, multiple Rave accounts can be merged into a single account. Thus the primary UUID referencing a user may change over time |
Account state |
A user can be in one of three account states: anonymous, personalized, or authenticated. See Player Account States for more information. |
Username |
A unique username in the Rave system. It can be changed, but at any given time it must be unique. Given that it can be changed, it should not be used in place of the UUID to refer to users. It is intended for display purposes. |
Display name |
A non-unique display name for the user |
Profile image URL |
A URL for the user’s profile image |
User’s email address |
|
Birthdate |
User’s birthdate |
Gender |
User’s gender |
FB UIDs |
One or more Facebook UIDs can be associated with a user. |
Google UID |
The user’s Google UID if they have connected to Google. |
Devices |
A list of devices associated with the user |
Sessions |
A list of active sessions a user has. Each application/device combination where a user is using Rave requires an active session |
Merged identities |
A list of user accounts that have been merged in to this user account. See Merged IDs for more information. |
User State Transitions#
A player’s Rave ID can change on a specific device when any of the following is true:
The device is reset when the player was in the “Anonymous” state
The player logs in to an account that was previously created on another device. This transitions the user on the curent device from the anonymous state to the authenticated state and the anonymous account they had been using on this device is automatically merged in to the account that they logged in to. A list of merged anonymous Rave IDs for an account and can be accessed via the User API.
The player opens game the first time after his account was merged with another account and the primary Rave ID was generated on the other device. See Merging for more information.
Player Account Identifiers#
As the player continues playing Rave-enabled games, over time they can add more information about themself and build out their gamer profile. They can connect his Facebook and Google accounts, create a display name and a username, as well as add a profile picture.
Player Account States#
A player account will be in one of the following three states, which will affect their ability to use different Rave features.
Anonymous - The player hasn’t provided any information to identify their account
Personalized - The player shared personal information such as email, but didn’t associate any information to be able to securely identify himself in the game. Secure authenticating identities include Big Fish ID, Facebook and Google.
Authenticated - Player connected and logged in with one or more of these identities: Facebook, Google, Big Fish ID
Player Value Scopes#
It is possible to apply an application scope to a subset of a user’s attributes. For each application, the display name and profile image can be configured to either use global or app-scoped values. This behavior can be controlled with the User value scope setting for an application in the Rave portal (see the Configuring an Application section).
When the App scope option is selected for an application, the following behaviors are enabled:
Display names and profile images that are set in the application are only visible in that application
Display name and profile image values displayed in the application will either be the app-scoped values, if set, or the global values if no app-scoped values have been set.
If a user removes an app-scoped value that they have set, the value displayed in the application will become the global value.
When the default scope Global is selected, display name and profile image are shared across all other apps that have Global scope selected.
Merging#
As of Rave SDK v2.9, complete player account merging is supported.
When a user is logging in to apps and games using different social networks on different devices, the user will be identified initially as different distinct users and as a result, there will be multiple Rave IDs defined for this user. As Rave observes the associations between this user’s social networks, it will collect a list of the identities for this unique user by way of merging them under a single Rave ID.
Example: Bob logs in to a game on his iPad using Facebook. Later, Bob logs in to a different game on his Android device using Google. At this moment, Bob has 2 different accounts (one for the Facebook login on one device, another for the Google account on his other device). Now Bob decides to request some gifts from his friends using Facebook on his Android device which he had only logged in to using Google. At this point, Rave is able to identify that Bob has used two accounts and Bob’s accounts are now merged into a single identity with the previous identity stored safely and without loss. Bob’s single Rave account is unified and for all future logins, he will be identified under the one unique account.
How Merging Works#
When an anonymous or personalized user logs in to an existing Rave account, or any user connects to an existing Rave account while already authenticated, Rave will merge all data (social data such as friends list and game data such as leaderboard scores) from the currently logged in user to the existing Rave account of which the user is trying to connect or login as. The result is a single Rave ID which has all of the combined associations with social networks and combined data from both accounts. To the user, it will simply appear as though the login or connect action worked without issue.
Rave maintains the list of all Rave IDs merged into the current account. See Merged IDs for information.
The merge function is triggered when RaveSocial.connectTo is used to connect the current user using authentication data already associated with another Rave user account. The result of the merge is a single user which maintains the previous identity as well as a combined set of both user’s data. This feature is enabled by default. To disable this feature, disable the Rave setting RaveSettings.General.AutoMergeOnConnect.
In a user merge case, the source user (currently logged in) is merged into the target user (existing Rave user matching connect credentials). The source user is then logged out after the merge is complete and the newly merged-in-to target user is logged in. The currently logged in Rave ID will switch from the source user to the target user. Any devices logged in with the source user will also switch to the target user automatically. Any devices logged in as the target user will be unaffected except for the data updates.
Merged IDs#
Rave creates a new Rave ID for every new anonymous player session when the setting RaveSettings.General.AutoGuestLogin is enabled. When an anonymous player subsequently logs in via, for example, Facebook, the Rave ID from the anonymous session is merged into the authenticated account. Account merge also happens when a logged in player attempts to connect a social network account (ie. Facebook) that is already connected to another Rave ID. The account is merged and the other Rave ID is stored in the list of merged IDs for the player’s account.
Obtaining the list of merged identities is available via the API RaveUsersManager.fetchIdentities. Please refer to the platform SDK API documentation for more information on usage.
Merged Data#
When two users are merged together, the users data is also merged using the following rules:
Identity - The source user’s RaveID will be added to the target user’s identities.
Contacts - The target user will retain its contacts and add all contacts of the source user.
Facebook, Google and other associations - The target user will add the associations from the source user for all social networks.
Leaderboards - The target user will use the best high scores earned between both the source and target user.
Achievements - The target user will keep all unlocked achievements from both the source and target user.
Gifts - The target user will keep all gifts from both the source and target user.
Accessing the Current User#
Once you have successfully logged in you can access the current user
// to access the current user use the following snippet:
id user = RaveSocial.usersManager.current;
// to update the current user use the following snippet
[RaveSocial.usersManager updateCurrent:^(NSError * error) {
if (error) {
// handle error condition
} else {
// perform operations on [RaveSocial.usersManager current]
}
}];
// to access the RaveID and other values for the current user use the following snippet:
// access is also provided to the current user through RaveSocial
id<RaveUser> currentUser = RaveSocial.usersManager.current;
NSString * raveId = currentUser.raveId;
if (! [currentUser isGuest]) {
// perform some action if the user is or isn't a guest account
NSString * bfId = currentUser.thirdPartyId;
NSString * fbId = currentUser.facebookId;
// etc.
}
Finding Users#
Find a user by email
[RaveSocial.usersManager fetchUser:[RaveUserReference byEmail:email] callback:^(id<RaveUser> user, NSError *error) {
if (error) {
// handle error
return;
}
// view, request friend, follow, block, unblock, etc
}];
Find a user by username
[RaveSocial.usersManager fetchUser:[RaveUserReference byUsername:username] callback:^(id<RaveUser> user, NSError *error) {
if (error) {
// handle error
return;
}
// view, request friend, follow, block, unblock, etc
}];
Find a user by Rave ID
[RaveSocial.usersManager fetchUser:[RaveUserReference byRaveId:raveId] callback:^(id<RaveUser> user, NSError *error) {
if (error) {
// handle error
return;
}
// view, request friend, follow, block, unblock, etc
}];
Blocking Users#
Block a user
[RaveSocial.usersManager blockUser:userReference callback:^(NSError *error) {
if (error) {
// handle error
return;
}
}];
Unblock a user
[RaveSocial.usersManager unblockUser:raveId callback:^(NSError *error) {
if (error) {
// handle error
return;
}
}];
Get a list of blocked users
[RaveSocial.usersManager fetchBlockedUsers:^(NSOrderedSet *users, NSError *error) {
if (error) {
// handle error
return;
}
}];
Two-Factor Authentication#
Rave’s two-factor authentication system is set up to work automatically with google’s Authenticator application. Rave offer’s three interfaces to implement in the RaveTwoFactorAuthenticationManager
class.
RaveTwoFactorAuthenticationPolicy#
The two-factor autentication policy interface can be passed into the activation, deactivation, and challenge methods of the two-factor authentication manager class. It contains a single method to let Rave know what code the user entered from their authenticator application.
Attention
These policies are typically implemented and set at a native scene pack level, even when working with Unity projects.
In addition to the policies, two-factor authentication must be enabled for your application in the Rave portal for a user to be presented with a 2FA challenge when authenticating.
@protocol RaveTwoFactorAuthenticationPolicy <NSObject>
@required
/**
* This method provides a structure for customization of two-factor authentiction flow. This can be implemented and set for activation, deactivation, or challenging a user on login.
*
* When implementing a custom activation, deactivation, or challenge policy, you can override the default UI that is shown to the user.
* A default policy is provided that uses basic system UI for inputting the code they retrieve from the device.
*
* @param callback The UI should pass back the code the user enters in this callback for the manager to use.
*/
- (void)presentChallenge:(RaveTwoFactorAuthenticationCallback)callback;
@end
This policy can be implemented and passed into manager to show what UI is presented to the user when receiving a 2FA challenge, and allow them to enter a code that they can retrieve from their authenticator app.
There are three different policies that need to be set, and the same implementation can be used for all of them.
Activation Policy#
You can set the activation policy by calling:
[RaveSocial.twoFactorAuthenticationManager setActivationPolicy:[SomeActivationPolicy new]];
This activation policy is what will be presented to the user when they start the enableTwoFactorAuthentication
, which is initiated when the following is called.
[RaveSocial.twoFactorAuthenticationManager enableTwoFactorAuthentication:^(NSArray * _Nullable backupCodes, NSError * _Nullable error) {
if(error) {
// Handle error
return;
}
// Notify user to store backup codes in a safe spot.
}];
This does several things. First, it retrieves a link and secret that is used to deep-link into the Google authenticator application and add the account to the list, all the user has to do from here is make a note of the code and input it in the dialog when navigating back to your application. As it does this, it also opens the dialog that is configured in the RaveTwoFactorAuthenticationPolicy
that is set for the activation policy.
Deactivation Policy#
You can set the deactivation policy by calling:
[RaveSocial.twoFactorAuthenticationManager setDeactivationPolicy:[SomeDeactivationPolicy new]];
This deactivation policy is what will be presented to the user when attempting to remove 2FA from the users account, which is done via the following:
[RaveSocial.twoFactorAuthenticationManager disableTwoFactorAuthentication:^(NSError *error) {
if(error) {
// Handle error
}
}];
Upon entering the code, two-factor authenticaiton will be removed from the account.
Challenge Policy#
The challenge policy is responsible for UI that will be displayed to the user when attempting to authenticate after enabling two-factor authentication for the account. It can be set with the following:
[RaveSocial.twoFactorAuthenticationManager setChallengePolicy:[SomeChallengePolicy new]];
URL Handler#
There is also a URL handler interface that must be implemented to customize what is done when Rave receives a URL and secret for a user. The recommended flow is to open the URL in the native way, so that the user is directed to the Google authenticator application automatically. It can be encoded in a QR code as well, or allow for customization of UI to allow the user to navigate to the application themselves.
Friends and Followers#
Note
Friends and Followers functionality is currently under deprecation. Please contact the Rave Social support representative for additional info.
Rave Social has an advanced in-game social graph management system which makes it easy to establish connections between users to create a social graph around your apps. Rave supports both Friends (private, or two-way) and Followers (public, or one-way) relationship models, and the Friends lists and Followers lists can be configured to be app-specific, or shared across several apps.
Both relationship models use similar APIs and concepts but have key differences in how relationships are established and maintained.
Friends#
Friends are two-way relationships between users, which require one person to send a Friend request, and the other to accept that request to establish the relationship. A user can send a friend request to any other user and they may either accept or reject the request.
Followers#
Followers are one-way relationships between two users. A user can follow any other user without the requirement to first send them a request. It is possible for the other user to reciprocate and also follow the first user, but in a reciprocating following relationship, where two users follow each other, the outcome is still two independent relationships and not a Friend relationship. The only way to get a Friend, or two-way relationship is to use the Friends model.
Lists#
Every app in the Rave system can use one or more lists of Friends and Followers. By default Rave generates one list of Friends and one list of Followers at the time a Rave application is created. These lists are private to that app. Rave also creates global Friends and Follower lists, which are accessible to all of a publisher’s apps. A developer can create any number of custom lists on top of these four lists for their application. Every list can be either a Followers or Friends list, but not both. Lists are created in the Rave portal. Lists are independent - adding friends or followers for a user to a list does not add those relationships in any other list.
Scopes#
Each list is assigned a specific scope. Scope can be either Global or Application. A globally-scoped list is read-write permission across all applications for the publisher. Application-scoped lists are only accessible to a specific application. Other applications cannot access an application-scoped list that is not created for them.
Querying#
In order to get the most up to date list of relationships for a given list, each lists must be individually
synced from the server to the client via a call to update* in the associated feature manager in the Rave SDK.
To sync Friends, you would call the updateFriendsList method in RaveFriends.
Once a list is synced, it can be queried in a number of ways using the query builder methods provided by the feature
manager.
To start a query in a Friends list, you would call the friendsListQuery
method in RaveFriends, or
to start a query in a Followers list, you would call the followersListQuery
or followingListQuery
in
the RaveFollowersManager. Queries enable modern user experiences, such as autocomplete, partial data searches
and pagination.
Friends and Followers classes#
RaveFriends
/ RaveFriendsManager
- Provides all friends feature functions.
RaveFriend
- A friend
RaveFollowers
/ RaveFollowersManager
- Provides all follower/following feature functions.
RaveFollower
- A follower or followee
ListQueryBuilder
- A query builder which provides a list of friends or followers
SearchQueryBuilder
- A query builder which provides a search filter within a ListQueryBuilder
Friends and Followers examples#
Update list of friends#
[RaveSocial.friendsManager updateFriendsList:listKey callback:^(NSError *error) {
if (error) {
// handle error
return;
}
// handle the successful update of the list
}];
Query all friends in list#
[[RaveSocial.friendsManager friendsListQuery:listKey] get];
Query some friends in list#
This will query 5 friends starting at offset 10 in order of display name
[[[[RaveSocial.friendsManager friendsListQuery:listKey] orderBy:RaveRelationshipOrderByDisplayName] offset:10 limit:5] get];
Find user to request#
RaveUserReference* userReference = [RaveUserReference byEmail:@"myfriend@gmail.com"];
[RaveSocial.usersManager fetchUser:userReference callback:^(id<RaveUser> user, NSError *error) {
if (error) {
// handle error
return;
}
// send request, follow, block, etc
}];
Send friend request#
[RaveSocial.friendsManager sendFriendRequest:listKey reference:userReference callback:^(NSError *error) {
if (error) {
// handle error
return;
}
// success!
}];
Accept friend request#
[RaveSocial.friendsManager acceptFriendRequest:listKey friendId:raveId callback:^(NSError *error) {
if (error) {
// handle error
return;
}
// success!
}];
Reject friend request#
[RaveSocial.friendsManager rejectFriendRequest:listKey raveId:raveId callback:^(NSError *error) {
if (error) {
// handle error
return;
}
// success!
}];
Update list of followers#
[RaveSocial.followersManager updateFollowersList:listKey callback:^(NSError *error) {
if (error) {
// handle error
return;
}
// handle the successful update of the list
}];
Query followers#
[[RaveSocial.followersManager followersListQuery:listKey] get];
Follow user#
[RaveSocial.followersManager followUser:listKey reference:userReference callback:^(NSError *error) {
if (error) {
// handle error
return;
}
// success!
}];
Unfollow user#
[RaveSocial.followersManager unfollowUser:listKey raveId:raveId callback:^(NSError *error) {
if (error) {
// handle error
return;
}
// success!
}];
Account Conflict Resolution#
Attention
To avoid unwanted conflicts it is critical that each app in your portfolio uses a consistent business mapping with Facebook . If incorrectly configured users that could be identified as the same across your portfolio will be misidentified as different users potentially leading to account conflicts later.
There are two types of conflicts that can occur when attempting to attach credentials to a Rave account. In the simpler case where different credentials of the same kind are already associated with current account the user will be offered the opportunity to use the new credentials instead. In the case where the credentials are already associated with a different Rave account several things can happen depending on your integration:
The default configuration will prompt the user to contact support.
The next option is to configure RaveSettings.General.AllowForceDisconnect to true. This will prompt the user to confirm that the current credentials should be forcably connected to the current user and removed from the old user.
Finally if the integration has set a merge policy then it will give you the opportunity to let the user choose whether or not to merge the current user with the user that already has these credentials associated. If so the current user will be merged into the foreign user. This cannot be undone and has implications across your portfolio so please consider carefully how best to implement this policy.
Rave supplies a default merge policy with your scene pack that provides a user merge dialog that you can customize or replace but you must explicitly set the provided policy as the current policy. By default no policy is set so merging is disabled.
Transitioning from Rave ID as a Save Game/Cloud Storage ID#
In the past you may have used Rave ID as a means to index user data stored on your own servers. There are downsides to this approach that you may have encountered including Rave ID changing for the current user. To create a more stable environment for data indexing we have created Application Data Keys.
Save Game/Cloud Storage ID with App Data Keys#
AppDataKeys provides a stable index for a given Rave user and application giving you the same key under most scenarios regardless of account changes. In the typical scenario the user’s Rave ID would be the index and would remain stable over the life of the application. Common scenarios include:
* Server-stored savegame keys
* Cloud storage indexing
* Any application data which can be accessed via a RaveID
When a merge between two accounts that have active sessions for your application should happen AppDataKeys provides an easy mechanism to resolve which key should be used to continue by giving your application a list of the possible index candidates. Your application can take that list, find indentifying information from your cloud storage and let the user choose or you can automatically chooose for them. For more information on AppDataKeys see Using App Data Keys for Save Game or Cloud Storage ID and AppDataKeys API.
Contacts#
Adding Friends from Contacts Providers#
As a user connects to a social network, like Facebook, Rave automatically finds your friends that use Rave. No additional integration work is necessary. In the particular case of Facebook, when the Rave client notices that your Facebook friends have changed it will update your Rave friends through the backend. All of this is taken care of for you automatically once you have successfully logged in using Facebook, or some other authentication provider. A user’s phonebook will also automatically be searched for Rave friends when they are first logged in.
Rave Social’s contacts API allows the developer to manage a list of contacts associated with the current user and interact with contacts from all supported social media sources as well as the local device phone book.
Automatic Syncing#
Rave will opportunistily update contacts based on a few settings and emergent SDK usage. Upon completion of connect, if RaveSettings.General.AutoSyncFriends includes the pluginKeyName for the social provider you’re using then, your contacts will sync automatically. Otherwise automatic syncing will be performed when updating contacts caches no more often than the time interval specified by RaveSettings.General.AutoSyncInterval.
RaveContact#
This class implements a single Rave contact. A Rave contact is stored on the server and can be retrieved using the
RaveContactsManager
. The Rave API will attempt to associate a contact on the server with an existing Rave user.
Social networks associated with a Rave account will be used to automatically fetch the user’s friends. Those friends
that have an associated Rave account are added as contacts.
In addition to Rave contact stored on the server, a social network can also be used to temporarily fetch a list of the user’s friends. These are known as external contacts and are only available as a result set from a fetch operation. They are not sent or stored on the server, but can be used locally by the application.
Both Rave contacts and external contacts contain the following information, if available:
RaveId
- Unique identifier for the user within the Rave API. If the Rave backend server is able to, it will
associate contacts with existing Rave users. If the contact is an external contact, this function will return null
DisplayName
- A contact’s chosen display name. Usually obtained from the source of the contact (FB, Google, etc…)
PictureURL
- A URL to the contact’s profile picture
Source
- A list of external sources where the contact was found or imported from. Sources include Rave, Facebook,
Google, Phonebook, and Third Party
ThirdPartySource
- Name of the third party source this contact originated from. A third party source is typically
customer-specific, and outside the standard list of supported social networks
Key
- Unique identifier for the contact, even if the contact is an external contact and not associated with a Rave
user
RaveContacts#
This class provides static, easy to use references to all functions in the RaveContactsManager
.
RaveContactsManager#
This class implements all functions related to managing contacts in the Rave API. The manager stores contacts in a
local cache that can be retrieved using the get
family of functions. Before the local cache is used,
it should first be updated by retrieving information from the server using the update
family of functions. After
the first update, contacts information can be quickly retrieved from the cache and updated from the server only as
needed.
Contacts can be deleted from the Rave backend server and added in a few different ways:
Manually added by username. Username is any arbitrary string that is used to identify the contact. In other sources, the username can also be an email address.
Manually added by email, marked as a phone book contact. Source of contact is set to phone book.
Automatically sync contacts from the local phone book to Rave.
Automatically sync contacts from Facebook to Rave.
In addition to Rave contacts, the RaveContactsManager
can also “fetch” external contacts from Facebook, Google,
and third party plugins. This retrieves a list of contacts from the specified social network or third part,
but does not store them on the server. This list can then be used for sharing, recommending, and gifting.
For get
, update
, and fetch
, contacts can be filtered by All, Using current application, and Not using
current application.
Attention
As of Facebook Graph API 2.0, Facebook will only return friends whom are already using the current application instead of a full list of the user’s friends.
Common Contacts Examples#
List All Contacts in One Pass#
[RaveSocial.contactsManager updateAll:^(NSError * error) {
if (error) {
// handle error
} else {
// process contacts through RaveSocial.contactsManager.all
}
}];
Accessing the Cached Contacts#
Most update
operations have a corresponding get
that returns cached data.
NSOrderedSet * cachedContacts = RaveSocial.contactsManager.all;
// process cached contacts
Similarly you can call getFacebook
/updateFacebook
, allUsingThisApplication
/updateAllUsingThisApplication
to update your Facebook friends and friends who are using this application respectively.
Getting a Random Selection of Contacts From the Game Community#
[RaveSocial.usersManager fetchRandomUsersForApplication:appUuid callback:^(NSOrderedSet * users, NSError * error) {
if (error) {
// handle error condition
} else if (users.count > 0) {
for (id<RaveUser> profile in users) {
// display the user
NSString * displayName = profile.displayName;
// save the username for later
NSString * username = profile.username;
// potentially call addContactByUsername later
}
}
}];
Adding a Contact#
[RaveSocial.contactsManager addContactsByUsername:@[username] withCallback:^(NSError *error) {
if (error) {
// handle error condition for adding the contact
} else {
// handle the successful addition of a contact
}
}];
Removing a Contact#
[RaveSocial.contactsManager deleteContact:raveId withCallback:^(NSError * error) {
if (error) {
// handle error condition for removing the contact
} else {
// handle the successful removing of a contact
}
}];
Fetch Contacts and Exclude Users Not Using the Current Application#
Fetch a list of contacts from all attached social networks and filter them by users already using the app, then process each contact differently depending on if they are a Facebook or a Google contact.
[RaveSocial.contactsManager fetchAllExternal:RaveContactsFilterIncludeOnlyUsingApp withCallback:^(NSArray *contacts, NSError *error) {
if (!error) {
for (id<RaveContact> contact in contacts) {
if (contact.isFacebook) {
// Process Facebook external contacts
} else if (contact.isGoogleIdentity) {
// Process Google external contacts
}
}
}
}];
Login and Cross-App Login#
Rave makes it easy to provide a little-to-no-typing login experience for players of Big Fish games.
A game developer can integrate a built-in Big Fish branded Player Login experience (see Player Login Experience for details), or use Rave’s Login APIs for directly logging into authentication providers like Facebook, BFID and Google and create a completely custom login experience. See Directly Logging In to Authentication Providers section for details on the APIs
Once the player logs into one of Big Fish’s games on a given device, Rave will automatically log the player into other games. In order to do this Rave, stores some information about the player’s identity on the device and enables each subsequent Big Fish game the player opens on the device to access that information to seamlessly log the player into the game.
Logging out in any game, and reopening the game doesn’t cause the player to automatically log in.
For testing purposes Rave SDK provides a CAL (cross app login) tool as part of the SDK package. This tool allows the person testing the game and Rave SDK integration to use the tool to delete the CAL data from the phone, therefore disabling auto login functionality. Explicitly logging into any app recreates the CAL data, and stores it in the same place.
For more information on the CAL tool, see the Troubleshooting section.
Achievements#
Rave Social’s achievements API allows the developer to retrieve and unlock achievements associated with the current game and user. Achievements are defined using the Rave portal.
Note
In order to use achievements in your application, you must define your achievements in the Rave Developer Portal. See the Achievements section in the portal documentation for instructions on how to create acheivements.
RaveAchievement#
This class implements a single Rave achievement, containing information about the achievement and the current user’s progress on it (locked or unlocked). The following fields are stored and accessible with a RaveAchievement
:
Key
- A unique key identifying the achievement
Name
- A display name used when showing the achievement
Description
- A longer form description of the achievement
ImageUrl
- A URL to the icon or image representing this achievement
Unlocked
- Whether or not the current player has unlocked (completed the requirements for) this achievement
RaveAchievements#
This class provides static, easy to use references to all functions in the RaveAchievementsManager
.
RaveAchievementsManager#
This class implements all functions related to retrieving information about achievements in the Rave API. The manager stores achievements in a local cache that can be retrieved using the “get” family of functions. Before the local cache is used, it should first be updated by retrieving information from the server using the “update” family of functions. After the first update, achievements information can be quickly retrieved from the cache and updated from the server only as needed.
Common Achievements Examples#
List All Achievements#
[RaveSocial.achievementsManager updateAchievements:^(NSError * error) {
if (error) {
// handle error
} else {
// process contacts through RaveSocial.achievementsManager.achievements
}
}];
Accessing the Cached Achievements#
Most update operations have a corresponding get that returns cached data
NSArray * cachedAchievements = RaveSocial.achievementsManager.achievements;
// process cached achievements
Mark an Achievement as Unlocked#
Unlocking an achievement indicates that the current user has completed all requirements to obtain the specified achievement. An achievement is referenced by a string of its unique key
[RaveSocial.achievementsManager unlockAchievement:achievementKey withCallback:^(NSError *error) {
if (error) {
// handle error condition for marking the achievement as unlocked
} else {
// handle the successful marking of an achievement as unlocked
}
}];
Leaderboards#
The Rave Social leaderboards system allows for various ways to organize and access high score data for your users. The system is designed to not access the server until you tell it to update, caching the last accessed data until then for optimal performance that is customizable to any use case or connectivity situation. Note that when in anonymous mode, you cannot submit scores to leaderboards and leaderboards will not display your scores in them. Also, when you request adjacent scores for a user that doesn’t have a score you will receive data as if their score is the worst possible.
Note
In order to use leaderboards in your application, you must define your leaderboards in the Rave Developer Portal. See the Leaderboards and Tournaments / Timed Leaderboards section in the portal documentation for instructions on how to create leaderboards.
Leaderboards Concepts#
Sorter - The order of a given Leaderboard amongst other Leaderboards. The leaderboard designated “1” for Sorter will be shown first in a list of leaderboards, etc.
Is Ascending - sort order of what the best score is. Used to determine if a new score is best.
High Score - score for this leaderboard
Global Position - ranking over all users
Friends Position - ranking over just the user’s group of friends
Tournaments, or Timed Leaderboards#
Leaderboards can be reset on a given interval, such as hourly, daily, weeklu and monthly. They can also start and end on a given pre-defined schedule.
For information on how to configure a leaderboard reset interval and start and end date, see the Rave Developer Portal, Leaderboards and Tournaments / Timed Leaderboards section.
iOS Leaderboard Examples#
You will need to import the following headers to use the RaveSocial Leaderboard Manager
#import <RaveSocial/RaveSocial.h>
#import <RaveSocial/RaveLeaderboardManager.h>
Updating and Getting Leaderboards#
You can work with leaderboards and their scores after updating them at least once from the server. It is up to you how often you want to ask the server for updates beyond the first time. After the first call, even if the app is closed and reopened, cached information will be used until the next update request.
[RaveSocial.leaderboardsManager updateLeaderboards:^(NSError * error) {
if (!error) {
for (id<RaveLeaderboard> leaderboard in RaveSocial.leaderboardsManager.leaderboards) {
// do something
}
// you can also get leaderboards by using a given key after updating
id<RaveLeaderboard> specificLeaderboard = [RaveSocial.leaderboardsManager getLeaderboardByKey:@"myLeaderboardKey"];
}
}];
Submitting Scores to a Leaderboard#
[RaveSocial.leaderboardsManager submitScoreByKey:@"myLeaderboardKey" withScore:@234042 withCallback:^(NSError * error) {
if (!error) {
//the score is submitted
}
}];
Getting the High Score and Position#
Before you can examine your score you will have to update the leaderboard for which you want detailed information:
[RaveSocial.leaderboardsManager updateLeaderboardByKey:@"myleaderboardKey" withCallback:^(NSError * error) {
if (!error) {
// check updated leaderboard details
}
}];
You can easily get the high score and your ranking in a leaderboard: either globally or amongst your friends
id<RaveLeaderboard> leaderboard = [RaveSocial.leaderboardsManager getLeaderboardByKey:@"myLeaderboardKey"];
NSNumber* highScore = leaderboard.highScore;
NSNumber* globalPosition = leaderboard.globalPosition;
NSNumber* friendsPosition = leaderboard.friendsPosition;
Note: these values will be null if you have not submitted a score
Getting Lists of Scores#
Since leaderboards for games can contain thousands of entries, there are various ways to get subsections of a given leaderboard. Each method has two versions: the global scores version and the scores just amongst your friends
Getting Scores by Page#
You can get scores in pages by specifying the page you want and the number of entries you want in each page. You can access the scores after calling the proper update function.
// for global scores
[RaveSocial.leaderboardsManager updateGlobalScoresByKey:@"myLeaderboardKey" withPage:@1 withPageSize:@15 withCallback:^(NSError * error) {
if (!error) {
NSOrderedSet* setOfScoreObjects = [RaveSocial.leaderboardsManager getGlobalScoresByKey:@"myLeaderboardKey" withPage:@1 withPageSize:@15];
}
}];
// for scores amongst friends only
[RaveSocial.leaderboardsManager updateFriendsScoresByKey:@"myLeaderboardKey" withPage:@1 withPageSize:@15 withCallback:^(NSError * error) {
if (!error) {
NSOrderedSet* setOfScoreObjects = [RaveSocial.leaderboardsManager getFriendsScoresByKey:@"myLeaderboardKey" withPage:@1 withPageSize:@15];
}
}];
Getting Scores by Nearby Page#
You can get the page of scores closest to the user’s high score
// for global scores
[RaveSocial.leaderboardsManager updateMyGlobalScoresByKey:@"myLeaderboardKey" withPageSize:@15 withCallback:^(NSError * error) {
if (!error) {
NSOrderedSet* setOfScoreObjects = [RaveSocial.leaderboardsManager getMyGlobalScoresByKey:@"myLeaderboardKey" withPageSize:@15];
}
}];
// for scores amongst friends only
[RaveSocial.leaderboardsManager updateMyFriendsScoresByKey:@"myLeaderboardKey" withPageSize:@15 withCallback:^(NSError * error) {
if (!error) {
NSOrderedSet* setOfScoreObjects = [RaveSocial.leaderboardsManager getMyFriendsScoresByKey:@"myLeaderboardKey" withPageSize:@15];
}
}];
Getting Scores Adjacent to the User Score#
You can get the scores nearest to a given user’s high score, after you call the update function
// for global scores the 15 scores before and 15 scores after the user, if available
[RaveSocial.leaderboardsManager updateMyGlobalScoresAdjacentByKey:@"myLeaderboardKey" withAdjacent:@15 withCallback:^(NSError * error) {
if (!error) {
NSOrderedSet* setOfScoreObjects = [RaveSocial.leaderboardsManager getMyGlobalScoresAdjacentByKey:@"myLeaderboardKey" withAdjacent:@15];
}
}];
// for scores amongst friends only, 15 before and 15 after the user, if available
[RaveSocial.leaderboardsManager updateMyFriendsScoresAdjacentByKey:@"myLeaderboardKey" withAdjacent:@15 withCallback:^(NSError * error) {
if (!error) {
NSOrderedSet* setOfScoreObjects = [RaveSocial.leaderboardsManager getMyFriendsScoresAdjacentByKey:@"myLeaderboardKey" withAdjacent:@15];
}
}];
Getting Scores For Leaderboard From Previous Reset Intervals (ie. last week’s tournament)#
For leaderboards that have a defined Reset Frequency you might want to access the scores from a previous reset period
// Build request. Set version to the leaderboard version you wish to retrieve.
RaveLeaderboardsRequest *request = [[[[[[[RaveLeaderboardsRequestBuilder builder] key:@"myLeaderboardKey"] page:@1] pageSize:@50] version:@1] update:YES] build];
[RaveSocial.leaderboardsManager getLeaderboardScores:request withCallback:^(NSOrderedSet *scores, NSError *error) {
// Update UI with new scores.
}];
Gifting and Requests#
The Rave Social gifting system allows for players across social environments to interact in a tangible way that can either be used as just a social tool or as a monetizable or incentivized feature in your game. Players can request a gift from another player, which gives that player an opportunity to send back a gift. Gifts may be accepted or rejected by the recipient.
Gifting Concepts#
Gift Type - Any conceptual thing a user can give to another (life, heart, money, etc)
Gift Request - A request from one user to another asking for a gift
Gift - An instance of a gift type which can be accepted or rejected by the recipient
-.. note:: - In order to use gifts or requests in your application, you must define your gifts that can be given or requested in the Rave Developer Portal. See the Gifts section in the portal documentation for instructions on how to create gifts.
Gifting and Request APIs#
You will need to import the following headers to use the RaveSocial Gift Manager
#import <RaveSocial/RaveSocial.h>
#import <RaveSocial/RaveGiftsManager.h>
Get Gift Types#
[RaveSocial.giftsManager updateGiftTypes:^(NSError * error) {
if (!error) {
// let the user choose between different gift types
for (id<RaveGiftType> giftType in RaveSocial.giftsManager.giftTypes) {
// do something
}
}
}]
// you can also get gift types by their specific key
id<RaveGiftType> giftType = [RaveSocial.giftsManager getGiftTypeByKey:@"myGiftTypeKeyKey"];
Get Gifts#
You can get the sender and type of each gift you’ve received and let the user determine if they want to claim or reject the gift.
[RaveSocial.giftsManager updateGifts:^(NSError * error) {
if (!error) {
for (id<RaveGift> gift in RaveSocial.giftsManager.gifts) {
// do something with each gift
}
}
}];
You can get specific gift objects if you already have its id.
id<RaveGift> aGift = [RaveSocial.giftsManager getGiftById:theGiftId];
Send Gift#
You can send a gift to other users of the same game, with a limit of 20 users per send call. This example sends a life to another player.
id<RaveUser> recipient = aUser; // fill in with the user you wish to send the gift to
[RaveSocial.giftsManager sendGiftsWithKey:@"life" toUsers:@[recipient] withCallback:^(NSArray * succeeded, NSArray * failed, NSError * error) {
if (!error) {
// do something with the error
}
if (succeeded.count > 0) {
// do something if the gift was accepted
}
if (failed.count) {
// do something for the failure case
}
}];
You can bulk send gifts using the gift’s key as well. You can send to a list of users, user IDs, or contacts. Note that in the list of contacts version the users can be on external social networks like Facebook.
[RaveSocial.giftsManager sendGiftsWithKey:@"myGiftTypeKey" toUsers:myArrayOfUsers withCallback:^(NSArray * succeeded, NSArray * failed, NSError * error) {
...
}];
[RaveSocial.giftsManager sendGiftsWithKey:@"myGiftTypeKey" toUsersById:myArrayOfUserIds withCallback:^(NSArray * succeeded, NSArray * failed, NSError * error) {
...
}];
[RaveSocial.giftsManager sendGiftsWithKey:@"myGiftTypeKey" toContacts:myArrayOfUsers withCallback:^(NSArray * results, NSError * error) {
...
}];
Accept/Reject Gift#
You can accept or reject gifts
id<RaveGift> gift = aGift; // fill in with the gift the user is responding to
// to accept the gift perform the following operation
[RaveSocial.giftsManager acceptGift:gift withCallback:^(NSError * error) {
// handle response or ignore it
}];
// to reject the gift peform the following operation
[RaveSocial.giftsManager rejectGift:gift withCallback:^(NSError * error) {
// handle response or ignore it
}];
// you can also accept/reject gift by gift id using acceptGiftById/rejectGiftById
Get Gift Requests#
You can get the requester and type of each gift request you’ve received and let the user determine if they want to grant or ignore the gift request.
[RaveSocial.giftsManager updateGiftRequests:^(NSError * error) {
if (!error) {
for (id<RaveGiftRequest> gift in RaveSocial.giftsManager.giftRequests) {
// do something with each gift request
}
}
}];
You can get specific requests if you already have its ID.
id<RaveGiftRequest> aGiftRequest = [RaveSocial.giftsManager getGiftRequestById:theRequestId];
Send Gift Request#
You can request a gift from other users of the same application with a limit of 20 users per send call. This examplifies requesting a life from another player.
id<RaveUser> recipient = aUser; // fill in with a player chosen by the user
[RaveSocial.giftsManager requestGiftWithKey:@"life" fromUsers:@[recipient] withCallback:^(NSArray * succeeded, NSArray * failed, NSError * error) {
if (error) {
// handle error
}
if (succeeded.count > 0) {
// do something if the gift request was accepted
}
if (failed.count) {
// do something for the failure case
}
}];
//there are also versions of these functions to use arrays of userIds instead of arrays of users
Grant/Ignore Gift Request#
You can grant or ignore(reject) gift requests.
id<RaveGiftRequest> giftRequest = aGiftRequest; // fill in with the gift request the user is responding to
// to grant the gift request perform the following operation
[RaveSocial.giftsManager grantGiftRequest:giftRequest withCallback:^(NSError * error) {
// handle response or ignore it
}];
// to reject the gift request peform the following operation
[RaveSocial.giftsManager ignoreGiftRequest:giftRequest withCallback:^(NSError * error) {
// handle response or ignore it
}];
//there are also versions of these functions to use request ids instead of request objects
Chat#
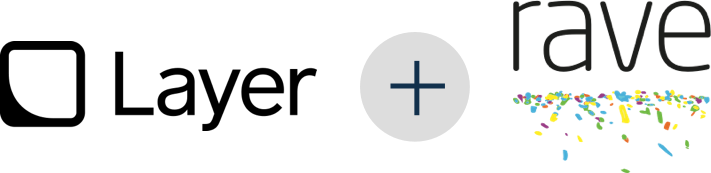
About Rave and Layer Integration#
Rave Social offers chat services with an out-of-the-box integration with Layer. Sign up for the Layer add-on to allow your users to send and receive messages, subscribe to chat channels and conversations, and view user online/offline statuses.
How it Works#
Let your Rave Account Manager know that you’d like to sign up for the Layer add-on
We configure your Layer app for you and ensure the link between your Layer and Rave applications
We will provide you a Layer URL to add to your application’s Rave configuration files for the RaveSettings.Chat.LayerURL value.
That’s it. Rave SDK wraps Layer’s messaging APIs, so now that your Layer app is configured you can use the Rave SDK to add user to user messaging to your app.
For additional documentation see: https://docs.layer.com
Localization#
Resources can be localized for a region and language using standard ISO 639-1 codes. A localized resource is one that exists in a directory named with the language code or the language-region code pair, such as “en” or “en-us”. Beyond this, resources do not need to receive any special treatment, as any resource in a locale-specific directory will override the same resource in the default resource directory.
Creating Localized Content#
Localizing your project to other languages is done with JSON files.
In /resources/default/strings.json
you will see the default translation file. Here you can define what strings will
be displayed for any language that doesn’t have a more specific string in its own language. Usually this JSON is used
for English, but you can make this whatever you want your default language to be. The format for each row is:
"key" : "translated language string",
Let’s look at a few rows from our default version of this file:
"Create your account" : "Create your account",
"OK" : "OK",
"RSEnterEmail" : "Enter the email address you use for your account. We'll email you a temporary password. <a href='http://www.ravesocial.co/help/index.html?rn=password'>Need Help?</a>",
The text to the left of the colon shows the key of the translation. Usually this is the English term in our existing code and XML files. The text to the right of the colon shows what will be substituted. Since this is for our English speaking users, often the string is the same as the key. Note that RSEnterEmail is a key. This is showing how you can put a shorter string in your code that can be substituted with something much longer in the translation file.
Localization Example#
Now let’s create a translation of the application. To localize to a language, simply copy the strings.json file from
/resources/default to /resources/XX where XX is the ISO-639-1 code for the language that you are creating your
localization for. In this example, the localization is for German, which has the country code de
.
Now you can edit the value for each key to be translated as shown below:
"Create your account" : "Erstelle Dein Spielekonto",
"OK" : "OK",
"RSEnterEmail" : "Gib die E-Mail-Adresse ein, die Du für Dein Spielekonto verwendest hast. Wir werden Dir ein vorübergehendes Passwort schicken. <a href='http://www.ravesocial.co/help/index.html?rn=password'>Brauchst Du Hilfe?</a>",
Attention
Ensure that the last row in your JSON dictionary does not end with a comma. Strict JSON prohibits this.
When you build and run the application and set the target device’s language as German, any strings for which there are translated values will show up in German.
Upgrading the Rave SDK#
The Rave SDK will always preserve your current user’s RaveID and credentials on upgrade.
Version-specific Upgrade Notes#
v3.5.1 Upgrade Notes#
- Xcode 8 support
Added support for Xcode 8.
- App Data Keys
Added full support for App Data Key management.
v3.4.6 Upgrade Notes#
- New required plist entries:
Starting with iOS10 Apple requires new privacy values in the plist when using Contacts. These are required when using scene pack components for adding phonebook contacts. (See New in SDK 3.4.2: Required plist key/value pairs to account for iOS 10 changes)
v3.4.2 Upgrade Notes#
- New required plist entries:
Starting with iOS10 Apple requires new privacy values in the plist when using the Camera or Photo Library. These are required when using scene pack components for modifying profile picture. (See New in SDK 3.4.2: Required plist key/value pairs to account for iOS 10 changes)
- New CAL requirements:
Due to deprecation of certain UIPasteboard methods we are adding shared container based storage for CAL data. To limit exposure to variance in functionality, pasteboard function changed between iOS10 Beta5 and Beta6 for example, it is required to implement shared container support going forward. (See New in SDK 3.4.2: Required iOS setting when RaveSettings.General.AutoCrossAppLogin is enabled)
v3.1.1 Upgrade Notes#
NOTICE: Rave now integrates with Facebook SDK v4.x starting with version 4.6. When using FBSDK 4.x please use the new variations of +[RaveSocial initializeRave…] that include the launchOptions where possible. These can be passed from your applications implementation of -[UIApplicationDelegate application:didFinishLaunchingWithOptions:].
v3.01 Upgrade Notes#
NOTICE: Please refer to https://developers.facebook.com/docs/ios/ios9 for other considerations. Similarly, due to increased security constraints in iOS9, a network security exception may be needed in your project’s plist for the BigFish SUSI API to work correctly.
There are minor but sweeping changes to some basic methods in the RaveSocial API. If you’re using the scene pack as the front-end to your integration you should be largely unaffected. If, however, you’re using login and other APIs directly there are some important changes to consider.
More callbacks have been simplified to RaveCompletionCallback
. That means that success is represented by a nil
result for the error. User cancellation is now representated by an error with domain set to NSCocoaErrorDomain
and code set to NSUserCancelledError
. Notable instances where the callback changed are +[RaveSocial loginWith...]
, +[RaveSocial connectTo...]
, +[RaveSocial disconnectFrom...]
. A RaveCompletionCallback
callback was added to +[RaveSocial logOut]
.
Constants for referring to social network providers have been changed (e.g. RaveProviderNameFacebook
is now RaveConnectPluginFacebook
). Some methods that referred to providers in their name now refer instead to plugins to more accurately reflect the extensible nature of Rave (e.g. +[RaveSharing shareWith: viaPlugin:...]
).
NOTICE: New integration point. Please add a call to
+[RaveSocial handleURL]
to your app delegates openURL method. This is automatically handled for Unity integrations.
v2.8 Upgrade Notes#
Considerations for upgrading the Rave SDK for an iOS project should be minimal.
NOTICE: Both Google and Facebook SDKs have been updated to their respective latest versions as for July 2014.
RaveToastWidget
has been replaced withRaveToast
- References toRaveToastWidget
should be updated as necessary+[RaveGifts sendGiftWithKeyToContactsAndShareVia]
- Theinteractive
boolean parameter for this function has been removed. The functionality it provided has been made automatic.
Upgrading from SDK with IDFA-On to IDFA-Off#
Using Facebook Tokens From Previous Non-Rave Integration#
Rave will automatically find and use an active Facebook session on startup if the session was created using the default session storage in the Facebook SDK. If the session did not use default storage, Rave provides an API which can be used to set the token to use. When using the explicit token setting method, it should only be used once so an integrator should delete the token from local storage once handed off to Rave for initialization. Either method will result in an automatic login to Rave either for the existing user linked to the Facebook account or a new Rave user will be created and linked to the Facebook account.
Explicit Token Setting Method#
You can set an access token from custom storage as shown below:
[RaveFacebookLoginProvider createAndRegister];
[[RaveSocial.loginProviderManager providerByName:RaveConnectPluginFacebook] useTokenForRaveUpgrade:accessToken];
SDK Logging#
Rave Social SDK can be configured to use any log level at run-time via RaveSettings
, using the setting
RaveSettings.General.LogLevel
.
Quiet - No Logging - Default
Error - Only Errors
Warn - Warnings and Errors
Info - Info, Warnings and Errors
Verbose - Verbose Messages, Info, Warnings and Errors
Debug - Debug Messages and all others included
You may override the default value for debugging by changing the config.json, the application plist, or by calling:
[[RaveSettings sharedInstance] set:RaveLogLevel toString:customLogLevel];
SDK Troubleshooting#
Troubleshooting#
Logging into Facebook from system settings fails without error: Check your RaveSettings.Facebook.ReadPermissions setting. If you’ve overriden this and the permissions specified are incorrectly formatted or mispelled the Facebook SDK will fail without error!
Logging into Facebook from Safari returns cancelled error when user authorizes app: Make sure your application delegate calls [+RaveSocial handleURL:…]
Using the CAL tool#
The Rave iOS CAL (Cross App Login) Utility provides a way to view and clear data that the SDK uses to log a user in to multiple game installations on the device. There are multiple versions of data supported which allows for CAL between newer and older integrations of Rave. A single button allows for all versions of data to be totally cleared. Clearing the CAL data does not affect the local app data for any installed apps and any Rave-enabled app may re-create data once used again. Clearing CAL data is intended for use in a testing environment only, though it will also work with production data.
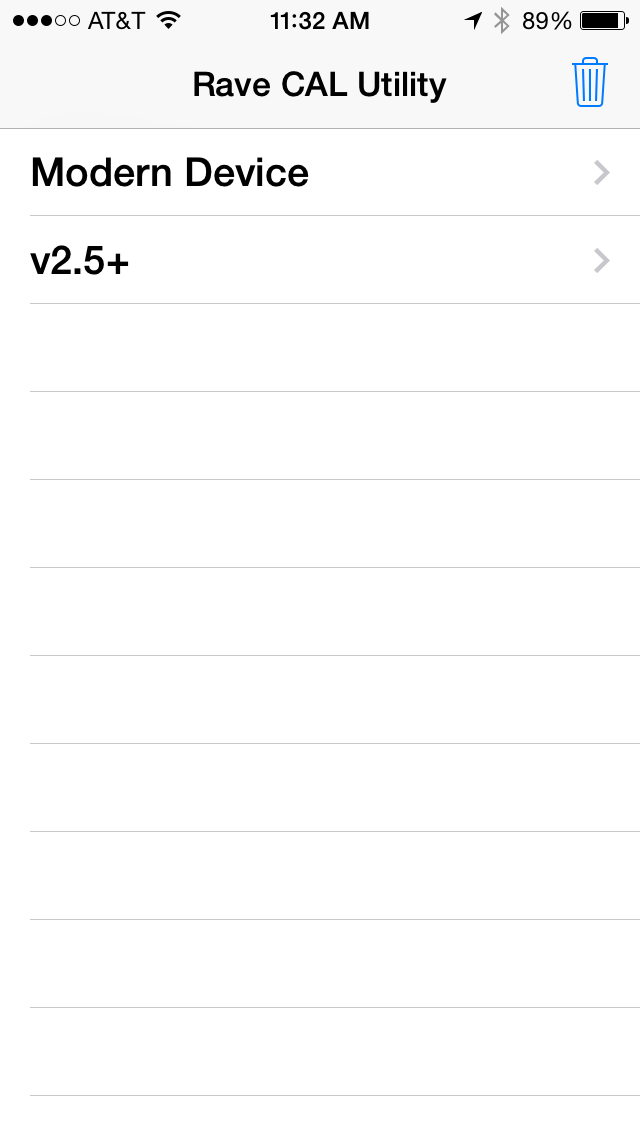
iOS CAL Utility screenshot
Note * Like CAL in general, you only have full functionality from this tool if it has been resigned with an identity from the same team that your app uses in Xcode.
Social Network State#
Once the player connects Facebook, Google, or the local Phonebook to his account, he will be able to use social features, such as posting to someone’s Facebook wall, directly from the game and from the built in Big Fish Rave personalization experiences. However, this will only be true if there is an authentication token present from the particular social network on the device from which the player was connecting. If the developer starts playing on another device, or wipes the current device, then the token will not be available and the player will have to log into Facebook again. The social network readiness API lets the developer know whether the usage of the social network is currently available for the player. See Checking Readiness Of Social Networks section for more details.