Player Login Experience#
The default Login experience allows the player to log in, and create a new gamer account via any of these methods:
Sign in with Facebook
Sign in with Google
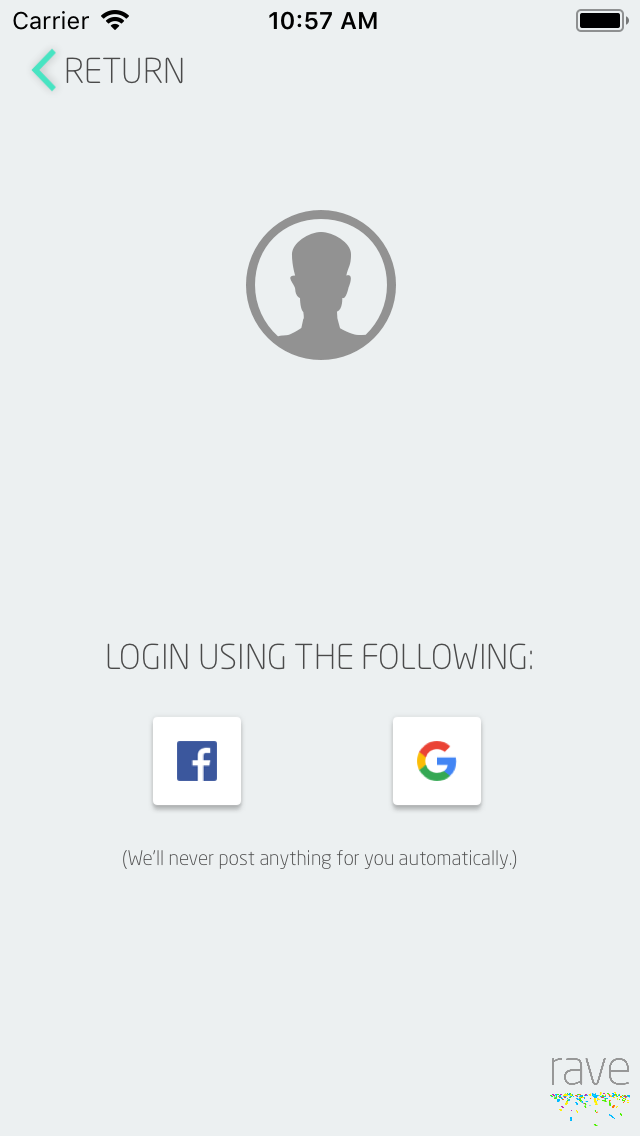
In order to integrate this scene it’s important that the Facebook and Google apps used in the Rave integration are properly configured. Please see Integrating Third Party Social Providers section for instructions on how to configure the social networks apps.
To integrate the Login experience:
RaveLoginScene * loginScene = [RaveLoginScene scene];
loginScene.didFinishWithRaveSocialCallback = ^(BOOL loggedIn, BOOL newUserCreated, NSString * pluginKeyName, NSError *error) {
if( newUserCreated ) {
NSLog(@"~~~it's a new user");
}
if( loggedIn ) {
NSLog(@"~~~login done");
}
else {
NSLog(@"~~~login cancelled");
}
};
[loginScene show];
Registering and Handling Login Callbacks#
RaveSocial provides key Scene and Login status callbacks that a developer can register and listen to.
Login Status Notification#
The general Login Callback (not to be confused with the RaveLoginScene
callback) is used to notify the developer
that a login status change of some kind has occured. This is useful because it may indicate that some social features
are now available.
[RaveSocial setLoginStatusCallback:^(enum RaveLoginStatus status, NSError * error) {
if (status == RaveLoginError) {
// respond to the error condition
} else if (status == RaveLoggedIn) {
// respond to loggedIn status
// at thish time you may also check different fields of the current user
// by accessing RaveSocial.usersManager.current
} else if (status == RaveLoggedOut) {
}
}];
Directly Logging In to Authentication Providers#
If using the built-in login experiences is not desired, developer can build completely custom login experience using Rave’s login APIs.
Using our loginWith
API, you can directly invoke a login via Facebook, or Google. Please use the following
constants:
@"Facebook"
@"Google"
[RaveSocial loginWith:@"Facebook" callback:^(NSError * error) {
// result handler
}];
Checking Readiness of Authentication Providers#
Just like with the direct login API - you can also see if an authentication provider is ready to be used. “Readiness” indicates
that a user has connected this social account to their Rave Social account and also that the token or local
authentication for this social account was successful. Call convenience functions in RaveSocial
to check a plugin’s
readiness. If a plugin is not ready but you desire a ready status, use the matching connect methods in RaveSocial
to establish a connection with the the desired plugin.
[RaveSocial checkReadinessOf:@"Facebook" callback:^(BOOL isReady, NSError * error) {
// handle result
}];
// connecting to Facebook if it fails a readiness check
[RaveSocial connectTo:@"Facebook" callback:^(NSError * error) {
if (error) {
// handle error
} else {
// success
}
}];
Player Profile Management Experience#
The profile management experience screen allows players to build out their game profile by adding a profile picture, email, and display name, as well as connecting various social networks to connect with friends.
To integrate the Profile Management experience:
RaveAccountInfoScene * accountInfoScene = [RaveAccountInfoScene scene];
accountInfoScene.didLogout = ^(BOOL stillLoggedIn, NSError* error) {
if( !stillLoggedIn ) {
NSLog(@"~~~they logged out");
}
};
[accountInfoScene show];