Tip
For the best development experience and compatibility, always use the latest version of Xcode.
Step 4: Add Required System Frameworks#
The Rave Social SDK depends on several Apple-provided frameworks. To add these to your project:
In the project navigator, select your project.
Select your app target.
Click on the General tab.
Scroll down to the Frameworks, Libraries, and Embedded Content section.
Click the + button.
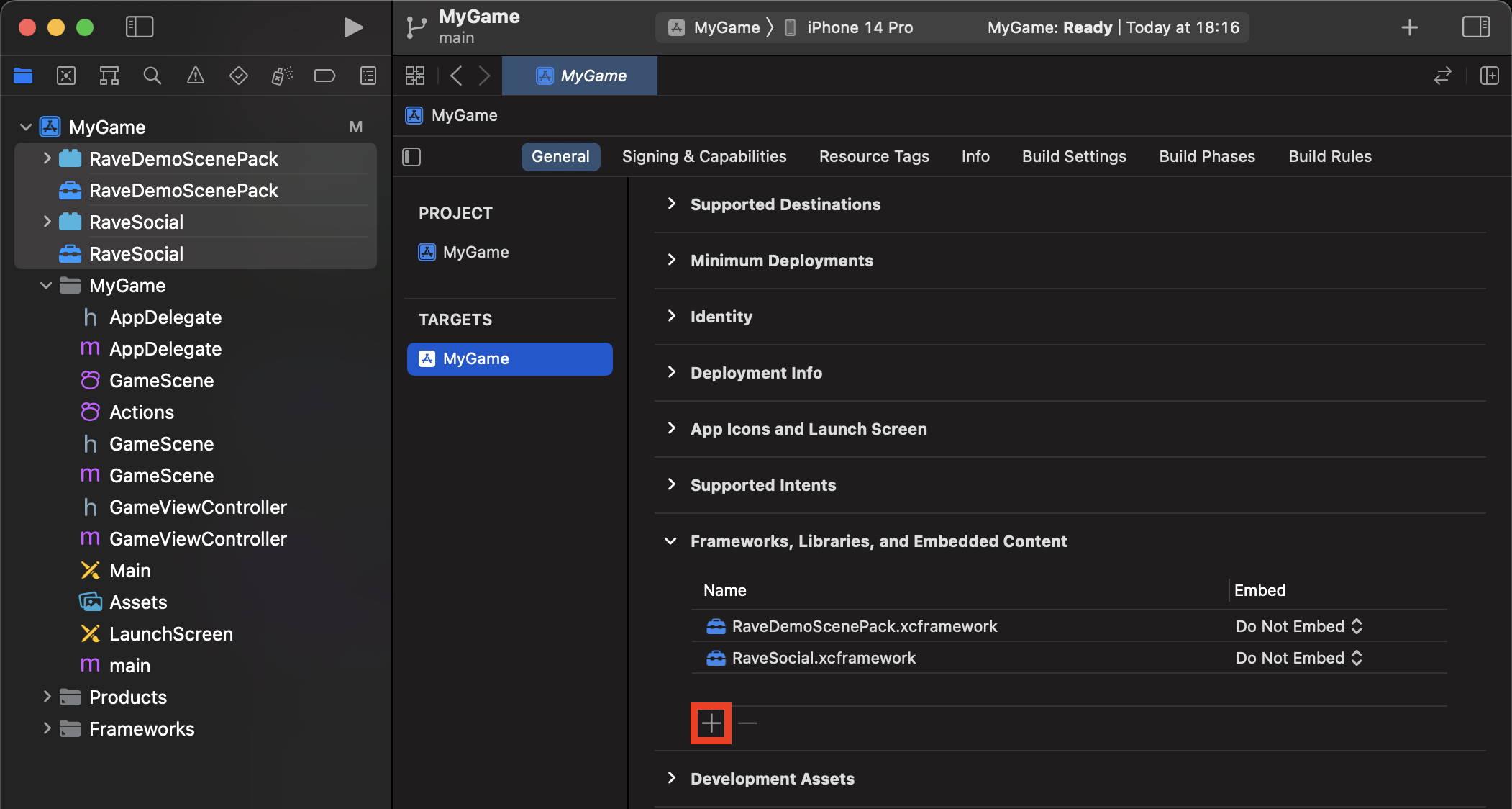
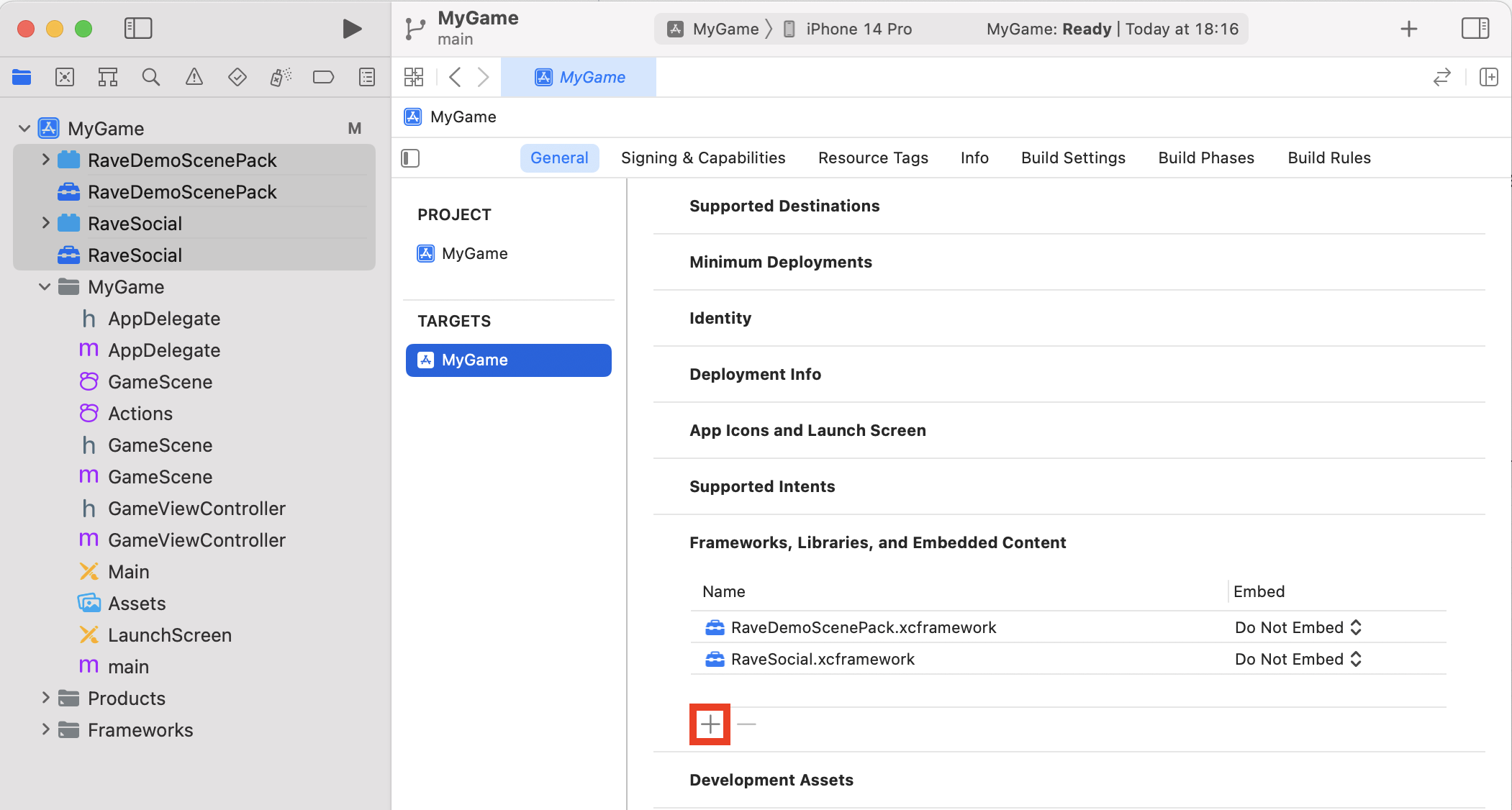
A view with your bundled frameworks will appear. Add the following libraries and frameworks:
Accounts.framework
AddressBook.framework
AdSupport.framework
AssetsLibrary.framework
CFNetwork.framework
CoreData.framework
CoreGraphics.framework
CoreLocation.framework
CoreMotion.framework
CoreText.framework
GameKit.framework
iAd.framework
libsqlite3.dylib
libxml2.dylib
libz.dylib
MediaPlayer.framework
MessageUI.framework
MobileCoreServices.framework
QuartzCore.framework
SafariServices.framework
Security.framework
Social.framework
SystemConfiguration.framework
Attention
Adding Third-Party Frameworks – If your app utilizes features that relies on third-party frameworks, you must ensure that they are included in your project. We recommend using a package manager such as CocoaPods to add these frameworks efficiently. CocoaPods simplifies the process of managing dependencies and ensures that all required libraries are correctly included in your project.
Here is a brief overview of the steps to add a third-party framework using CocoaPods:
Install CocoaPods if it is not already installed.
Set up CocoaPods in your project.
Add the required third-party frameworks to your Podfile.
Install the pods and open the generated .xcworkspace file in Xcode.
For detailed instructions on how to use CocoaPods, please refer to the CocoaPods Getting Started guide.
For integration with specific social platforms, add the following dependencies to your Podfile:
Google Sign-In: If your app utilizes Google Sign-In, add the following line to your Podfile:
pod 'GoogleSignIn'
Facebook SDK: If your app utilizes Facebook, add the following lines to your Podfile:
pod 'FBSDKCoreKit' pod 'FBSDKLoginKit' pod 'FBSDKShareKit'
Warning
Missing third-party frameworks can cause runtime crashes and integration issues. Double-check that all necessary frameworks are included in your project and are properly configured.
Step 5: Add Linker Flags#
Click the root project node in the “Project Navigator”
Click the “Build Settings” tab
Ensure “All” is selected
Search for “Other Linker Flags”
Double-click the right side of the table view row
Add
-ObjC
to the linker flags
Step 6: Edit Your Project’s .pch file#
Open your project’s .pch file (usually named ProjectName-Prefix.pch), and add the following to it:
#ifdef __OBJC__
#import <RaveSocial/RaveSocial.h>
#endif
Step 7: Edit Your Project’s Target Properties#
Add the following keys to your Project’s “Target Properties” in the “Info” tab with their corresponding values (see the demo for example usage):
FacebookAppID must be set to the appropriate value as the Facebook SDK internally refers to it by this name
FacebookClientToken must be set with the value found under Settings > Advanced > Client Token in your Facebook App Dashboard
The URLScheme for Facebook which must match the FacebookAppID provided in the JSON config (with “fb” prepended)
The URLScheme for Google Identity which must match the inverse of the Google client ID.
The URLScheme which must match the bundle ID for your app
Be sure your bundle identifier matches the identifier you register with Facebook, and Google
New in SDK 3.4.2: Required plist key/value pairs to account for iOS 10 changes#
NSContactsUsageDescription - String value will be shown when prompting user permission to access phonebook contacts (i.e. “Contacts will be used to find in-game friends”). If absent application will crash when attempting to access contacts
NSCameraUsageDescription - String value will be shown when prompting user for permission to access the camera (i.e. “Camera will be used to createa profile picture”). If absent application will crash when attempting to access the camera
NSPhotoLibraryUsageDescription - String value will be shown when prompting user for permission to access their photo library (i.e. “Photo library is used for profile pictures”). If absent application will crash when attempting to access the photo library
New in SDK 3.4.2: Required iOS setting when RaveSettings.General.AutoCrossAppLogin is enabled#
RaveSettings.IOS.ApplicationGroupIdentifier - identifier for application group to be used for sharing Cross-application Login data between apps. Must be application group specified in application entitlements for the integrating app. Required when Cross-application Login is enabled.
Step 8: UIApplicationDelegate Window Property Requirement#
UIApplicationDelegate
has an optional window property starting in iOS 5. The Rave Social iOS client uses this window
to display some overlays. Please ensure your application delegate implements the window property. Failure to ensure
window creation and access will result in a missing selector and the client will not be able to run.
// Declaring the property in your app delegate interface will cause
// Xcode to create the methods for you:
@property (nonatomic, strong) UIWindow * window;